Search Engine Optimization 12 Dec 2024
How to extend ASP.NET Core 3.0 and 3.1 Identity user
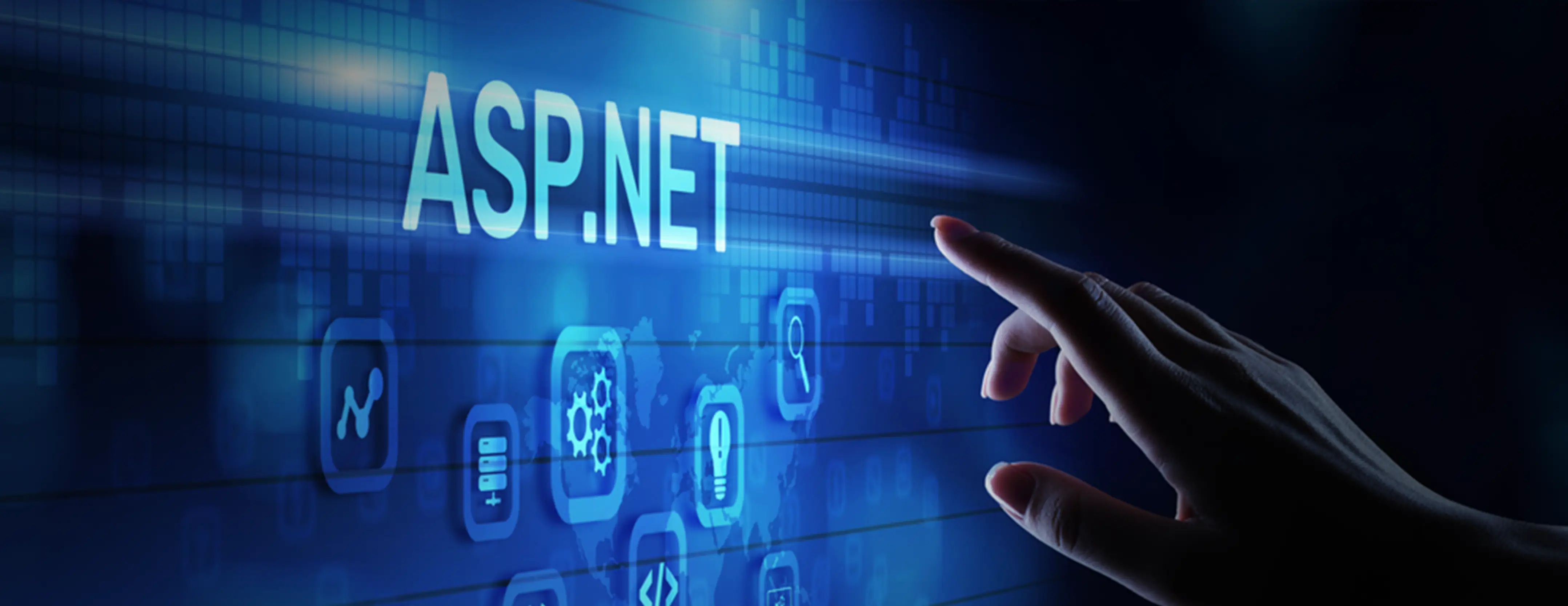
ASP.NET Core is a game changer special version 3.0 it's shipped with a lot of new features and performance improvements. so we decided to start using ASP.NET Core 3.1 for our new developments projects.
But when we try to extend identity user to display the user first name it didn't work as expected, and after some research we find a great article written by Adrien Torris about "How to extend ASP.NET Core Identity user" which work perfectly with asp.net core 2.2 but not with asp.net core 3.0.
we review the breaking changes for migration from asp.net core Version 2.2 to 3.0 and updated Adrien code to work with asp.net core 3.0 and 3.1.
so lets start with extending asp.net core 3.0 identity user
Using Microsoft.AspNetCore.Identity; public class ApplicationUser : IdentityUser { }
Using Microsoft.AspNetCore.Identity; public class ApplicationUser : IdentityUser { [PersonalData] public string FirstName { get; set; } [PersonalData] public string LastName { get; set; } }
- 3.add new class "ApplicationClaimsPrincipalFactory" that will Inherits from UserClaimsPrincipalFactory
public class AppClaimsPrincipalFactory : UserClaimsPrincipalFactory<ApplicationUser, IdentityRole> { }
public class AppClaimsPrincipalFactory : UserClaimsPrincipalFactory<ApplicationUser, IdentityRole> { public AppClaimsPrincipalFactory( UserManager<ApplicationUser> userManager , RoleManager<IdentityRole> roleManager , IOptions<IdentityOptions> optionsAccessor) : base(userManager, roleManager, optionsAccessor) { } }
using Microsoft.AspNetCore.Identity; using Microsoft.AspNetCore.Identity.EntityFrameworkCore; using Microsoft.EntityFrameworkCore; using Microsoft.Extensions.Options; public class AppClaimsPrincipalFactory : UserClaimsPrincipalFactory<ApplicationUser, IdentityRole> { public AppClaimsPrincipalFactory( UserManager<ApplicationUser> userManager , RoleManager<IdentityRole> roleManager , IOptions<IdentityOptions> optionsAccessor) : base(userManager, roleManager, optionsAccessor) { } public async override Task<ClaimsPrincipal> CreateAsync(ApplicationUser user) { var principal = await base.CreateAsync(user); if (!string.IsNullOrWhiteSpace(user.FirstName)) {
((ClaimsIdentity)principal.Identity).AddClaims(new[] { new Claim(ClaimTypes.GivenName, user.FirstName) }); } if (!string.IsNullOrWhiteSpace(user.LastName)) {
((ClaimsIdentity)principal.Identity).AddClaims(new[] { new Claim(ClaimTypes.Surname, user.LastName), }); } return principal; } }
public static class IdentityExtensions { }
using System.Security.Claims; using System.Security.Principal; public static class IdentityExtensions { public static string FirstName(this IIdentity identity) { var claim = ((ClaimsIdentity)identity).FindFirst(ClaimTypes.GivenName); // Test for null to avoid issues during local testing return (claim != null) ? claim.Value : string.Empty; } public static string LastName(this IIdentity identity) { var claim = ((ClaimsIdentity)identity).FindFirst(ClaimTypes.Surname); // Test for null to avoid issues during local testing return (claim != null) ? claim.Value : string.Empty; } }
- 8.now you can add display the First Name using razor syntax in the view by caling it's name FirstName()
@User.Identity.FirstName()